What is Express JS and Hello World in Express JS Framework
December 25, 2022 - by admin
Express.js is a web application framework for Node.js, a JavaScript runtime built on Chrome’s V8 JavaScript engine. It is designed to make the development of web and mobile applications in Node.js more efficient and easy. Express.js provides a robust set of features for web and mobile applications and is the most popular Node.js framework.
Express.js allows you to handle HTTP requests and responses in a more organized and efficient way. It provides a simplified interface for working with the Node.js HTTP server, making it easy to handle routing, middleware, and other common web application tasks.
One of the main advantages of Express.js is that it is lightweight and flexible. It allows developers to build web applications of any size and complexity, from small single-page applications to large enterprise-level systems.
Another advantage of Express.js is that it has a large and active community of developers. This means that there are many resources available to help developers learn and use the framework, including tutorials, documentation, and a wide range of third-party modules.
Express.js also has a middleware-based architecture, which makes it easy to add functionality to your application by using pre-built middleware or writing your own. This flexibility enables developers to build applications that are highly customizable and can be tailored to meet the specific needs of their business or organization.
Additionally, Express.js is compatible with a wide range of template engines and databases, which makes it easy to integrate with existing systems and technologies.
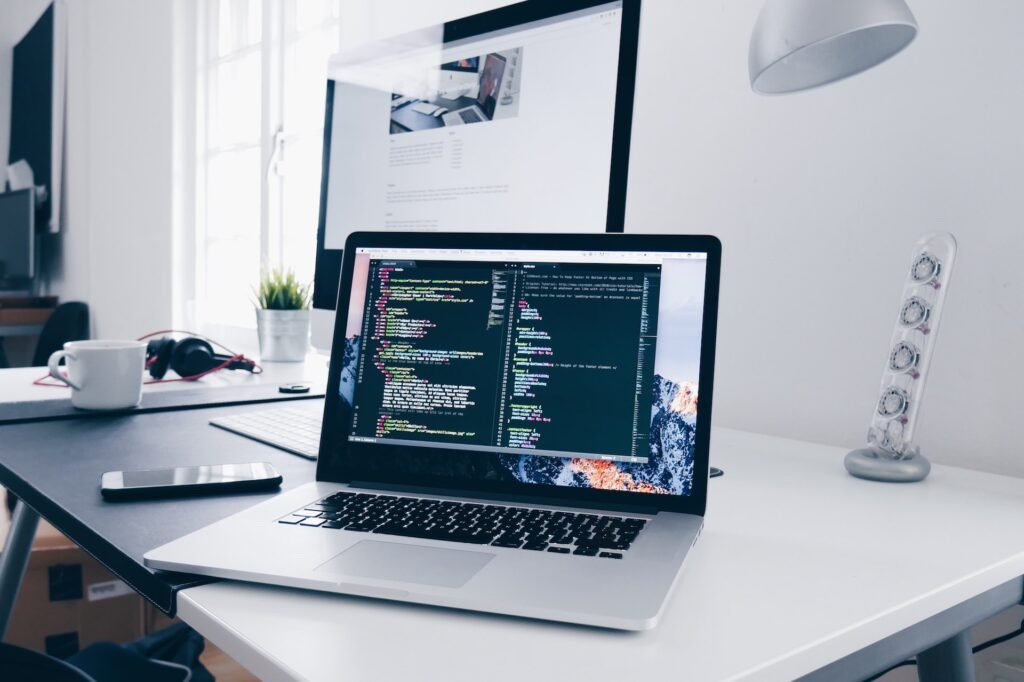
Using Express.js is relatively simple and straightforward. Here are the basic steps for getting started with Express.js:
- Install Node.js: Express.js is built on top of Node.js, so you will need to have Node.js installed on your computer before you can start using Express.js. You can download the latest version of Node.js from the official website (https://nodejs.org/en/)
- Create a new project folder and initialize it as a Node.js project by running the following command:
npm init
- Install Express.js by running the following command
npm install express
- Create a new file called “app.js” or “server.js” in your project folder, this file will be the entry point for your application
- In your “app.js” or “server.js” file, import the Express.js module by adding the following line at the top of the file:
- Run your application by typing the following command in your terminal or command prompt
node app.js
const express = require('express')
const app = express()
const port = 80
app.get('/', (req, res) => {
res.send('Hello World!')
})
app.listen(port, () => {
console.log(`Example app listening on port ${port}`)
})
This is the basic setup of an express application, you can start building your application by adding more routes, middlewares and other features. Additionally, you can also add other modules and packages to enhance the functionality of your application.
In conclusion, Express.js is a powerful, lightweight, and flexible web application framework for Node.js. It offers a robust set of features for web and mobile applications and has a large and active community of developers. Its middleware-based architecture and compatibility with a wide range of template engines and databases make it a great choice for building web applications of any size and complexity.