How to use jQuery Ajax in your web project
December 24, 2022 - by admin
AJAX stands for Asynchronous JavaScript and XML. It is a technique for creating fast and dynamic web pages. It allows for the exchange of data with a server without the need to refresh the entire page.
jQuery is a popular JavaScript library that makes it easy to use AJAX. To use jQuery’s AJAX functionality, you would use the jQuery.ajax() or one of its shorthand methods like jQuery.get() or jQuery.post().
Here is an example of how you might use jQuery’s .ajax() method to retrieve data from a server and update a section of a web page:
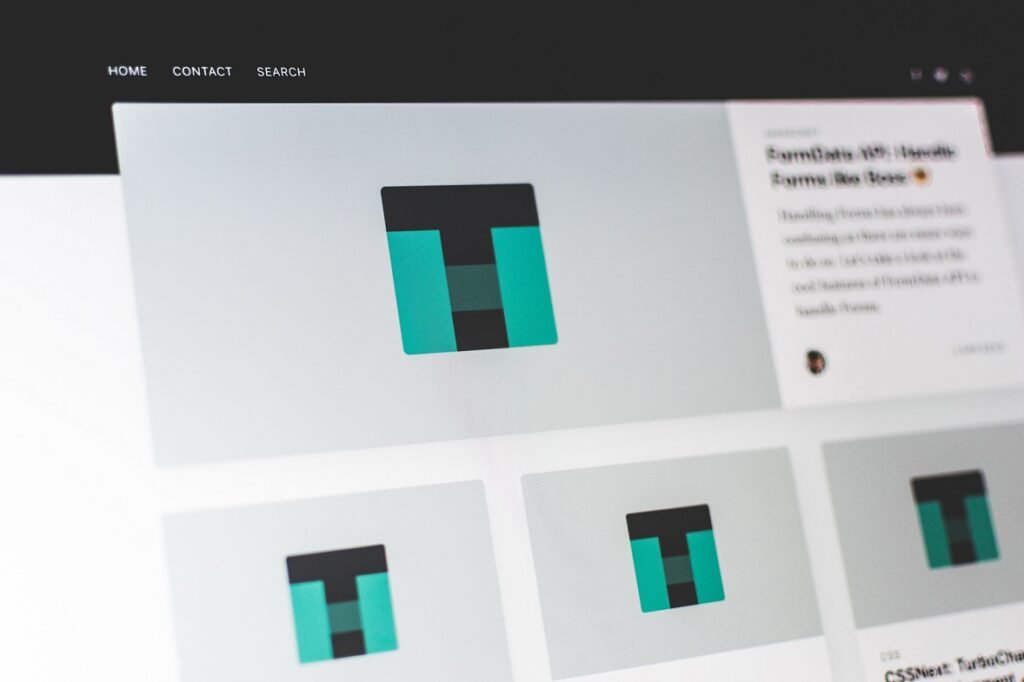
<!----------------- jQuery CDN ------------------>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.3/jquery.min.js" integrity="sha512-STof4xm1wgkfm7heWqFJVn58Hm3EtS31XFaagaa8VMReCXAkQnJZ+jEy8PCC/iT18dFy95WcExNHFTqLyp72eQ==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<script>
$(function() {
$.ajax({
url: 'get-user.php',
dataType: 'json',
type: 'post',
data: {id:1,action:'read'},
success: function( data, textStatus){
alert(data);
},
error: function( jqXhr, textStatus){
console.log(textStatus);
}
});
});
</script>
You can also use jQuery’s .get() or .post() method for a simpler way of making an ajax request
$.get( "your-url-here", function( data ) {
$( "#your-div-id-here" ).html( data );
});
$.post( "your-url-here", function( data ) {
$( "#your-div-id-here" ).html( data );
});
Note that the above examples are using the shorthand methods of ajax, you can also use the full ajax call with the same parameters, but with a little more verbose.
Additionally, you can pass data to the server, handle errors, and specify other options through the jQuery.ajax() method. You can find more information about jQuery’s AJAX functionality in the jQuery documentation at https://api.jquery.com/jquery.ajax/